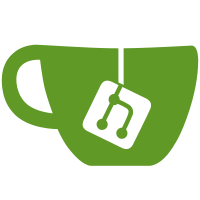
- Refactored package to match my site name - Renamed "logging" package to "stream" - Fixed various bugs - Fixed issues with illegal reflective access - Added proper throwable for when a dangerous threaded action happens in async - Added more reflective tools - Added ability to auto-generate simple wrapper classes - Added tools for converting enums to a flag-like system - Added annotation scanning for a supplied classloader - Properly renamed module to "libRefTools"
20 lines
684 B
Java
20 lines
684 B
Java
package net.tofvesson.reflection;
|
|
|
|
import java.lang.annotation.Annotation;
|
|
import java.util.ArrayList;
|
|
import java.util.List;
|
|
import java.util.Vector;
|
|
|
|
public class Annotations {
|
|
public static List<Class<?>> getAllAnnotatedClasses(Class<? extends Annotation> annotation){
|
|
@SuppressWarnings("unchecked")
|
|
Vector<Class<?>> classes = (Vector<Class<?>>) SafeReflection.getValue(annotation.getClassLoader(), ClassLoader.class, "classes");
|
|
ArrayList<Class<?>> a = new ArrayList<Class<?>>();
|
|
if(classes==null) return a;
|
|
for(Class<?> c : classes)
|
|
if(c.isAnnotationPresent(annotation))
|
|
a.add(c);
|
|
return a;
|
|
}
|
|
}
|