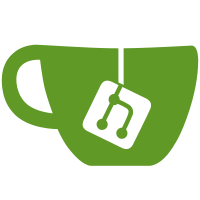
- Started creating a new revision of the ShiftingList - Populated entries are shifted to the end of the array - Shifting is done in blocks - Referring to indexes is more secure now - Added final collection helper class - Started adding ShiftingMap based on ShiftingList - Made SafeReflection helper class final
15 lines
377 B
Java
15 lines
377 B
Java
package com.tofvesson.collections;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.Collection;
|
|
|
|
@SuppressWarnings("unchecked")
|
|
public final class Collections {
|
|
public static <T> ArrayList<T> flip(Collection<T> c){
|
|
ArrayList<T> a = new ArrayList<T>();
|
|
T[] t = (T[]) c.toArray();
|
|
for(int i = c.size()-1; i>0; --i) a.add(t[i]);
|
|
return a;
|
|
}
|
|
}
|