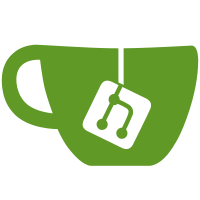
- Refactored package to match my site name - Renamed "logging" package to "stream" - Fixed various bugs - Fixed issues with illegal reflective access - Added proper throwable for when a dangerous threaded action happens in async - Added more reflective tools - Added ability to auto-generate simple wrapper classes - Added tools for converting enums to a flag-like system - Added annotation scanning for a supplied classloader - Properly renamed module to "libRefTools"
40 lines
1.2 KiB
Java
40 lines
1.2 KiB
Java
package net.tofvesson.async;
|
|
|
|
import java.lang.reflect.Method;
|
|
|
|
/**
|
|
* Pre-written anonymous class. Follows all naming conventions of an anonymous class, acts like an anonymous class and handles data like an anonymous class.
|
|
* It just isn't an anonymous class technically speaking...
|
|
*/
|
|
class EcoRunnable implements Runnable{
|
|
|
|
private final EcoAsync this$0;
|
|
private final Object val$o;
|
|
private final Method val$method;
|
|
private final Object[] val$params;
|
|
|
|
EcoRunnable(EcoAsync this$0, Object val$o, Method val$method, Object[] val$params){
|
|
this.this$0 = this$0;
|
|
this.val$o = val$o;
|
|
this.val$method = val$method;
|
|
this.val$params = val$params;
|
|
}
|
|
|
|
public void run() {
|
|
synchronized (this) {
|
|
try {
|
|
this$0.setLocal();
|
|
this$0.ret = val$method.invoke(val$o, val$params);
|
|
this$0.complete = true;
|
|
} catch (Throwable t1) {
|
|
if(!this$0.failed)
|
|
{
|
|
this$0.failed = true; this$0.t=t1;
|
|
}
|
|
} finally {
|
|
this$0.newThread(val$o, val$method, val$params);
|
|
}
|
|
}
|
|
}
|
|
}
|